For those of you who own Laravel-based websites, providing accurate and natural translation content is very important to translate your website and offer a good user experience.
Fortunately, several automatic translation services are available to help developers overcome this challenge, including Google Cloud AI API Translation and Google Translate. Both services offer translation capabilities using machine technology.
In this article, we will explore the main differences between implementing Google Cloud AI Translation and Google Translate for Laravel applications and recommend the best translation services for your Laravel website.
What are Google Cloud AI API translation & Google Translate?
Before entering into the discussion, we will discuss the respective meanings of Google Cloud AI API and Google Translate translations.
Google Cloud AI API translation
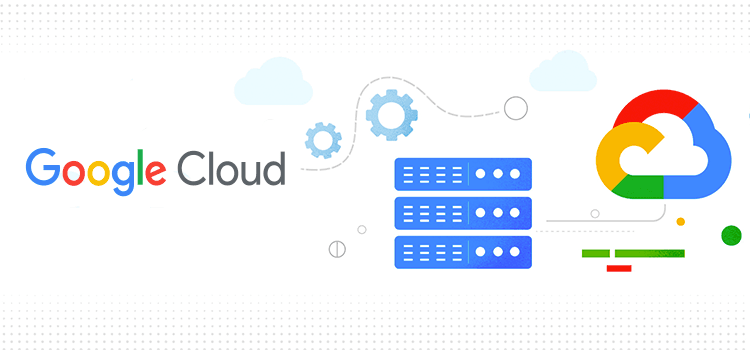
Google Cloud AI API Translation is an advanced automatic translation service provided by Google Cloud Platform. This service leverages the latest artificial intelligence (AI) and machine learning technologies to deliver accurate and natural translations of text, voice, and documents.
Google Cloud AI API Translation uses neural translation models based on deep learning, which allows it to capture language nuances and context better, providing more idiomatic translations that align with actual language usage. Additionally, using Google Cloud AI Translation for up to 500,000 characters is free of charge, while the next 75,000 characters will incur a cost of $20 per million characters.
Google Translate
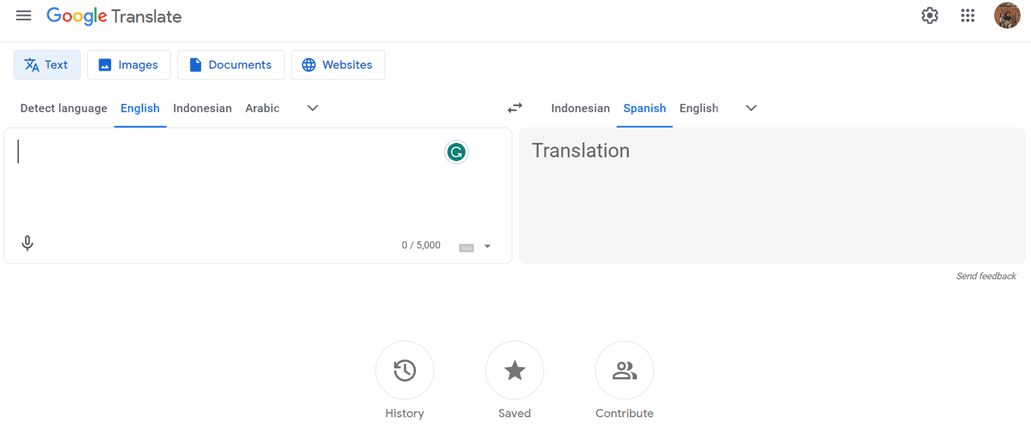
Meanwhile, Google Translate is a long-standing and widely known automatic text translation service. It uses a more traditional statistical translation approach, which generates translations based on patterns and probabilities learned from large training datasets.
Although it is not as advanced as Google Cloud AI API Translation in terms of accuracy and handling language nuances, Google Translate remains a popular choice due to its ease of use and free availability for non-commercial use.
Implement Google Cloud AI API translation on Laravel
Several translation services, including Linguise, use AI Translation cloud technology to translate websites.
The language translation service uses cloud AI API as one of its translation technologies. The following are the implementation steps on the Laravel website. We assume here that you already have a ready Laravel project website.
Create Linguise account
To use Linguise, you must register an account first. You can do this for free or subscribe for one month or a year. You only need to provide information such as your email, username, and password.
Add Laravel website info
After that, add information about the website you will use, enter your Linguise account info and web URL, select the Laravel platform, and add the default language and the language you want to add to the website.
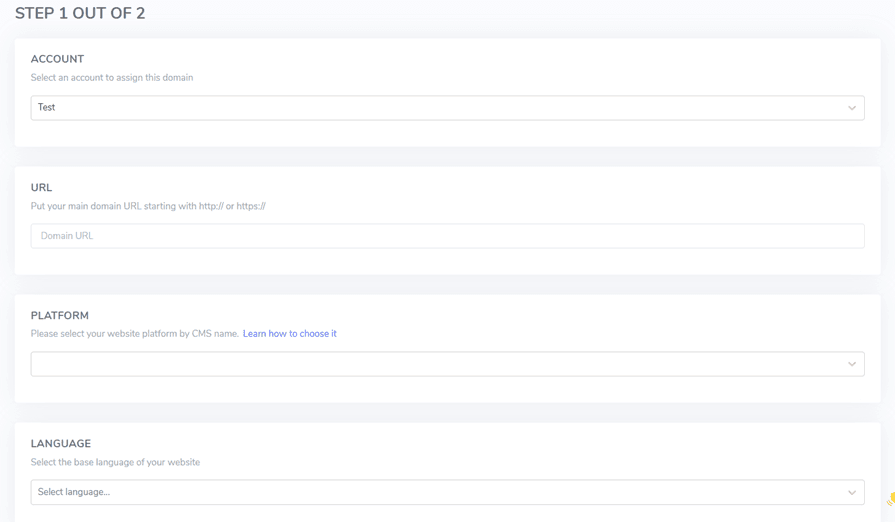
Then download the PHP script via the following button and save it on your local computer.
Upload and configure the PHP script
After downloading the script, unzip it and upload it to the root folder where Laravel is installed. Ensure it is located at the root of your Laravel installation (typically where your CMS files are). Ensure the folder is named “linguise” (the default name when the folder is unzipped).
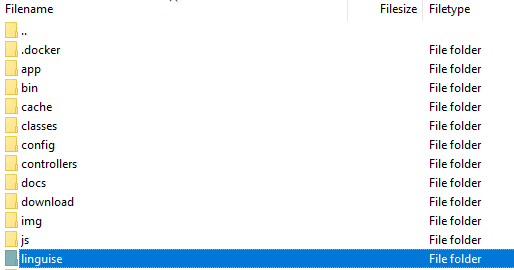
Then, the Linguise API key must be copied into the …/linguise/Configuration.php file you uploaded to your server. Edit the file and paste your API key between the quotes, replacing the text REPLACE_BY_YOUR_TOKEN.
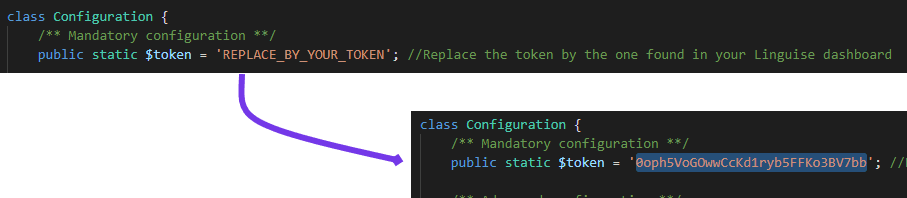
Insert script of language switcher
Next, insert the language switch script that you got from the Linguise dashboard.

Inserted into the front.blade.php file in the resources/views/layouts/ directory. Here is a preview of the inserted script.
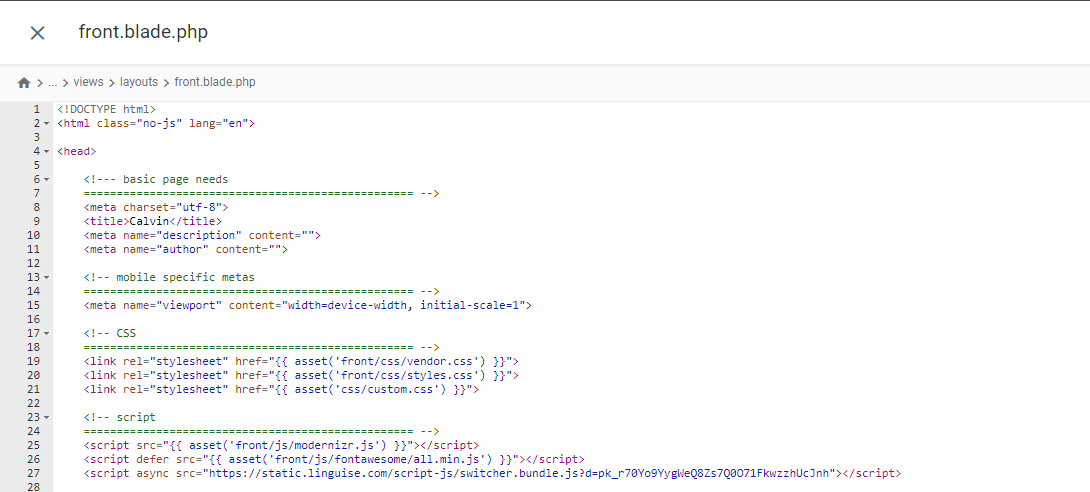
Configure .htaccess
Lastly, language-based URLs need to be configured in the .htaccess file. If your file includes “RewriteBase /,” simply copy and paste the following code after that line.
RewriteEngine On
RewriteRule ^(af|sq|am|ar|hy|az|eu|be|bn|bs|bg|ca|ceb|ny|zh-cn|zh-tw|co|hr|cs|da|nl|en|eo|et|tl|fi|fr|fy|gl|ka|de|el|gu|ht|ha|haw|iw|hi|hmn|hu|is|ig|id|ga|it|ja|jw|kn|kk|km|ko|ku|ky|lo|la|lv|lt|lb|mk|mg|ms|ml|mt|mi|mr|mn|my|ne|no|ps|fa|pl|pt|pa|ro|ru|sm|gd|sr|st|sn|sd|si|sk|sl|so|es|su|sw|sv|tg|ta|te|th|tr|uk|ur|uz|vi|cy|xh|yi|yo|zu|zz-zz)(?:$|/)(.*)$ linguise/linguise.php?linguise_language=$1&original_url=$2 [L,QSA]
Done. At this point, you have successfully implemented cloud AI API Translation on the Linguise service. Now, you can check the Laravel website. A default language switcher will appear, and you can customize the Language switcher for Laravel via the dashboard.
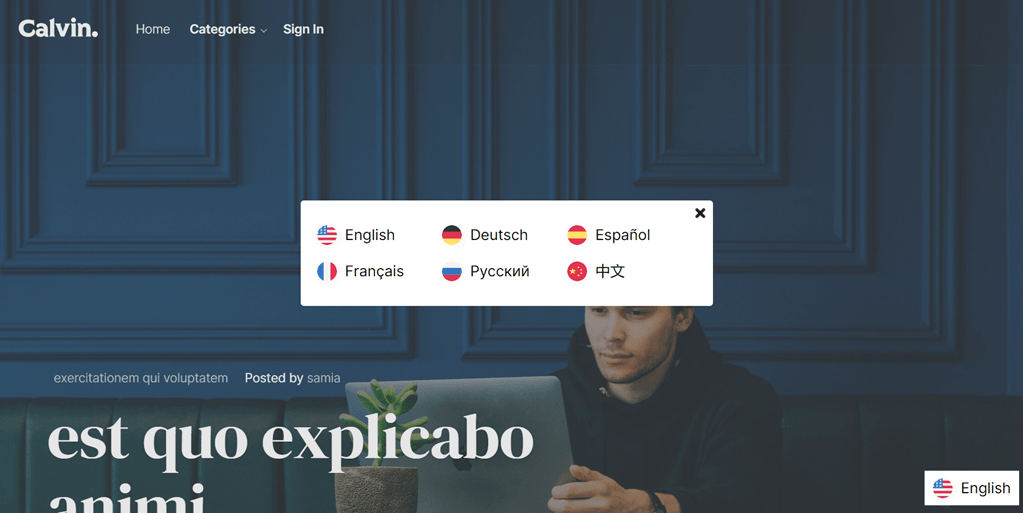
Implement Google Translate on Laravel
Now we will try to implement Google Translate in Laravel. Here we assume that you have successfully created a Laravel project and just need to add Google Translate.
You can also find the following installation steps on the official Laravel website.
Install Google Translator Package
To install the Google Translator Package, open the project prompt and input the following command. This package will enable you to utilize the working functions of the google-translator-language.
composer require stichoza/google-translate-php
Setting of Google Translator Package
We must set up the “google translator package” within the the application. Open the app.php file in the /config folder, find the “aliases” section, and add the following line of code.
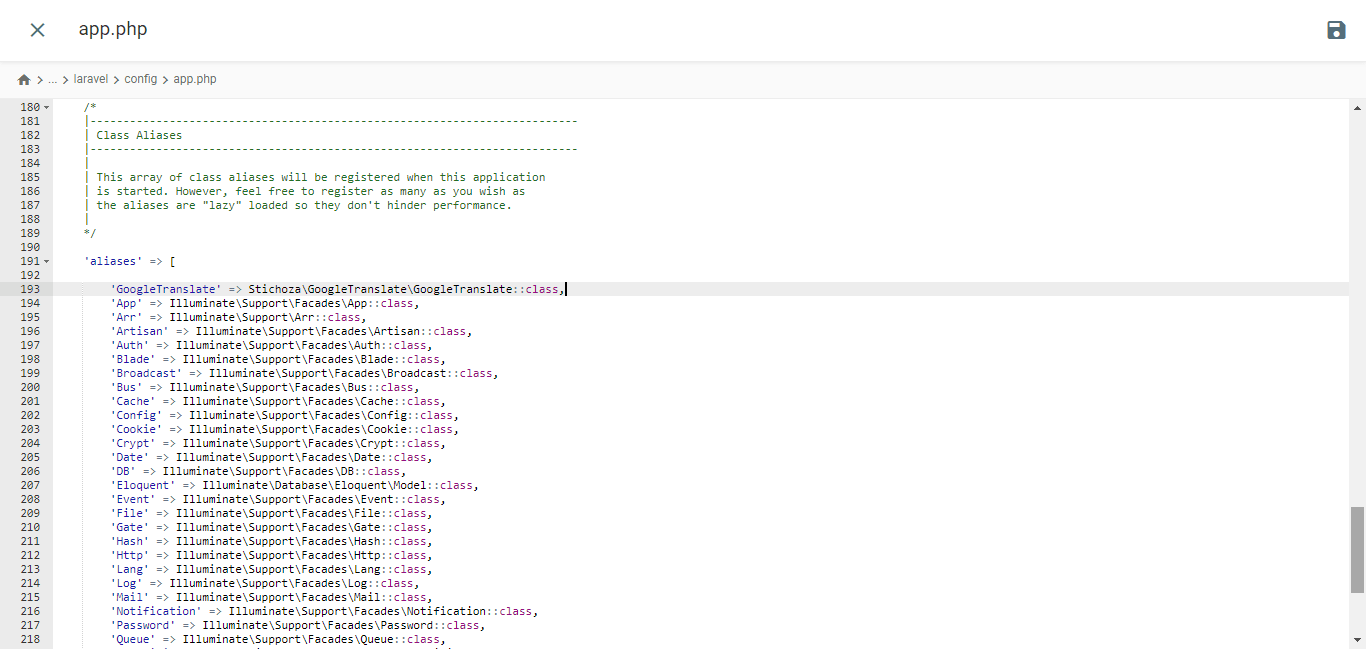
/*
|--------------------------------------------------------------------------
| Class Aliases
|--------------------------------------------------------------------------
|
| This array of class aliases will be registered when this application
| is started. However, feel free to register as many as you wish as
| the aliases are "lazy" loaded so they don't hinder performance.
|
*/
'aliases' => Facade::defaultAliases()->merge([
// 'Example' => App\Facades\Example::class,
'GoogleTranslate' => Stichoza\GoogleTranslate\GoogleTranslate::class,
])->toArray(),
Setup Language Controller
You need to create a language controller file like the following
php artisan make:controller LangController
It will create LangController.php file inside /app/Http/Controllers folder.
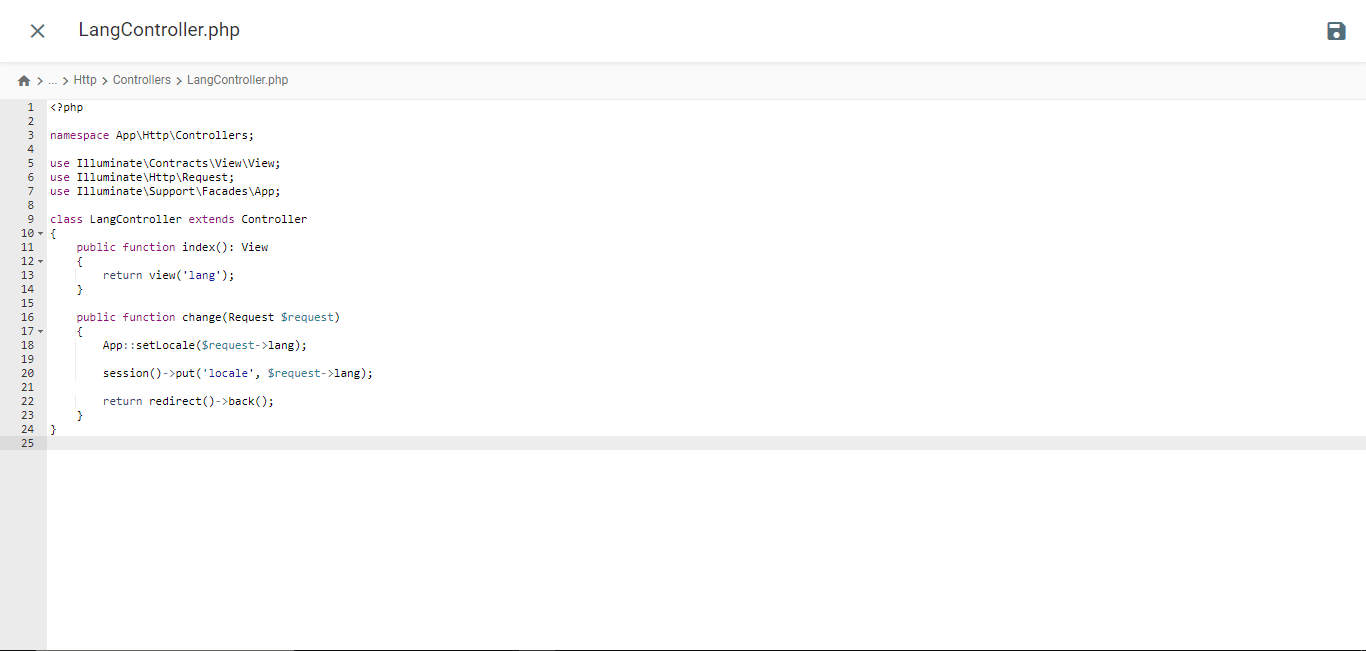
lang);
session()->put('locale', $request->lang);
return redirect()->back();
}
}
Create Blade Template File
Navigate to the /resources/views folder and create a file named lang.blade.php. Open the file and insert the following code into it.
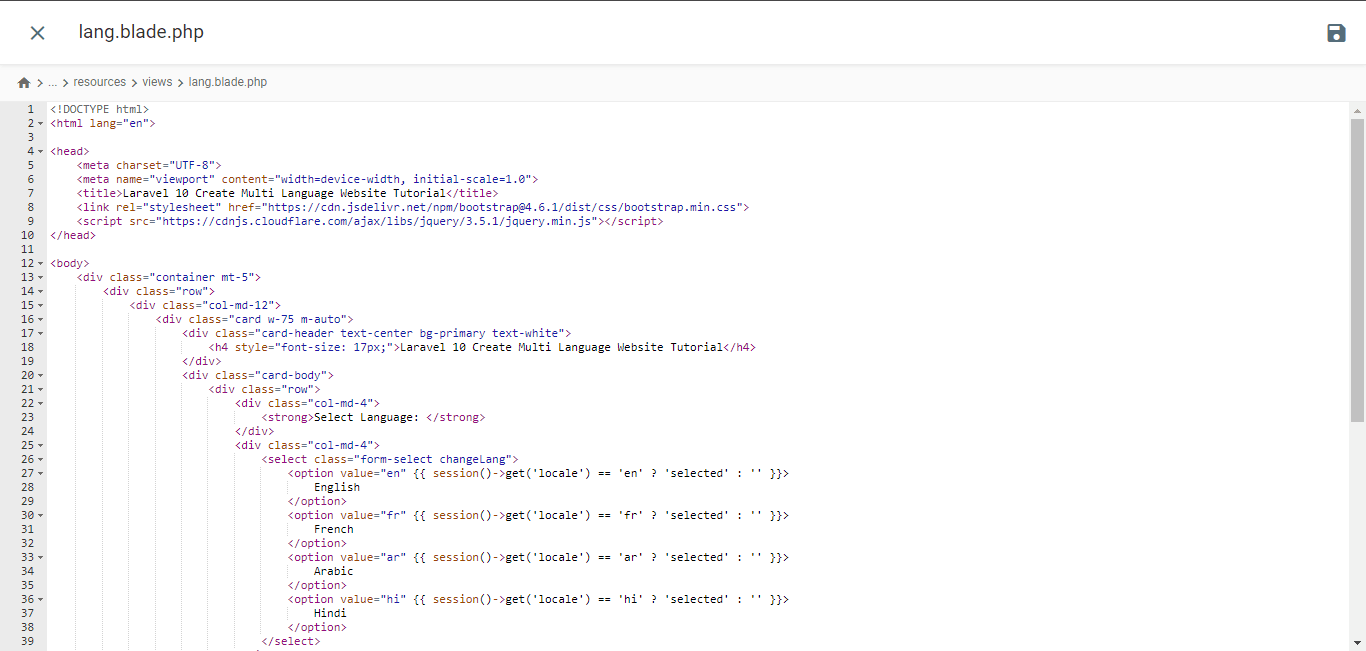
Laravel 10 Create Multi Language Website Tutorial
Laravel 10 Create Multi Language Website Tutorial
Select Language:
{{ GoogleTranslate::trans('Welcome to Online Web Tutor', app()->getLocale()) }}
{{ GoogleTranslate::trans('It is a passionate and innovative Web Development Community dedicated to empowering aspiring web developers with the latest tools and techniques. Our platform offers a comprehensive range of web development courses, including PHP and its frameworks, Node.js, MySQL, Javascript and WordPress.', app()->getLocale()) }}
Setting of Language Middleware
Open the project terminal and run this command,
php artisan make:middleware LanguageManager
It will create a file LanguageManager.php inside /app/Http/Middleware folder and paste this.
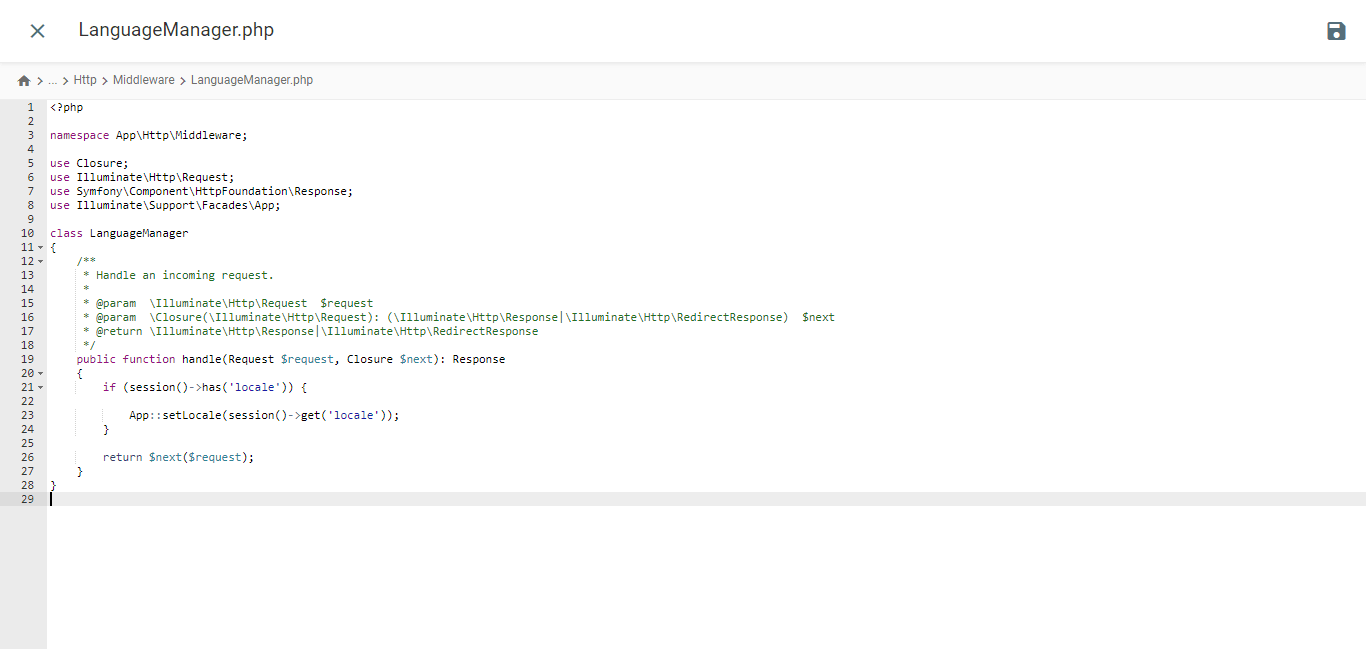
has('locale')) {
App::setLocale(session()->get('locale'));
}
return $next($request);
}
}
Register Language Middleware via Kernel.php
Open the Kernel.php file located in the /app/Http directory. Look for the $middlewareGroups array and navigate to the web section.
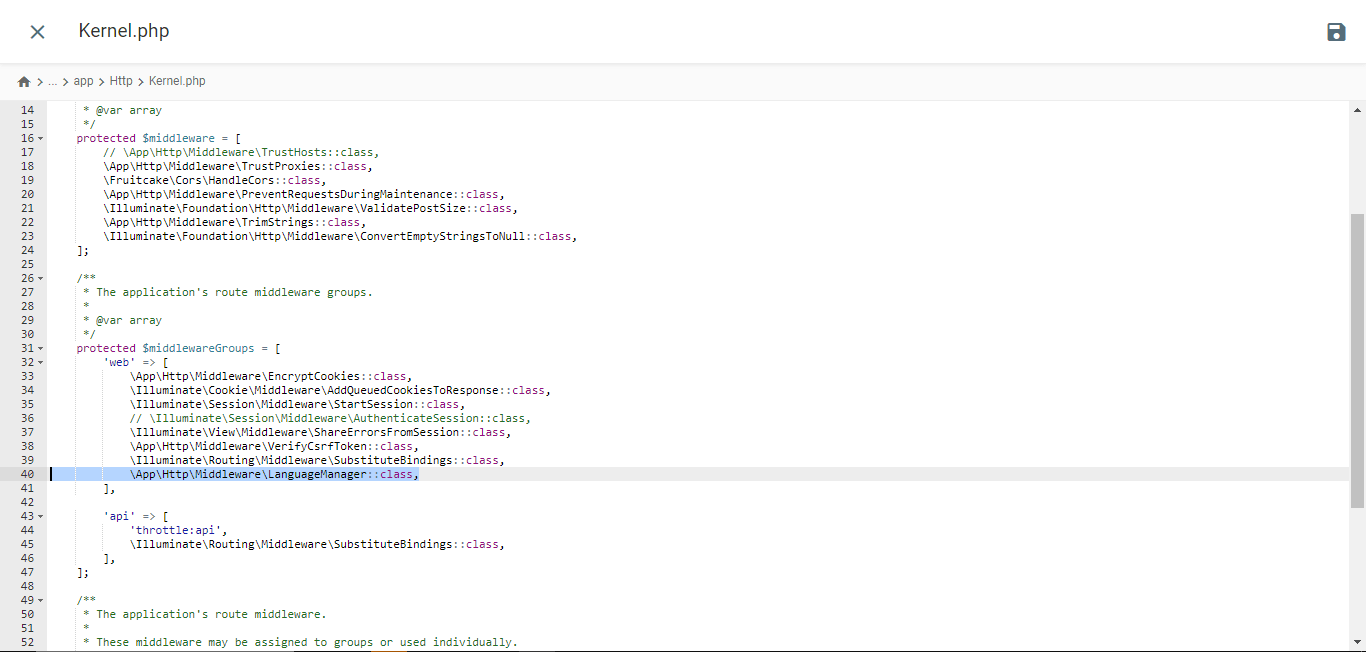
/**
* The application's route middleware groups.
*
* @var array>
*/
protected $middlewareGroups = [
'web' => [
//...
\App\Http\Middleware\LanguageManager::class,
],
'api' => [
//...
],
];
Add route
Open web.php from /routes folder and add these routes into it.
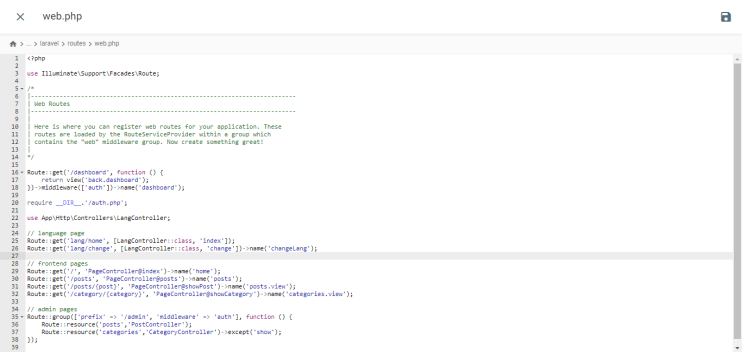
//...
use App\Http\Controllers\LangController;
//...
Route::get('lang/home', [LangController::class, 'index']);
Route::get('lang/change', [LangController::class, 'change'])->name('changeLang');
Testing website
Execute this command in the project terminal to launch the development server.
php artisan serve
Here’s a display of the Laravel website from website onlinewebtutorblog.com that has been created, as you can see in the following display there is a Google Translate dropdown.
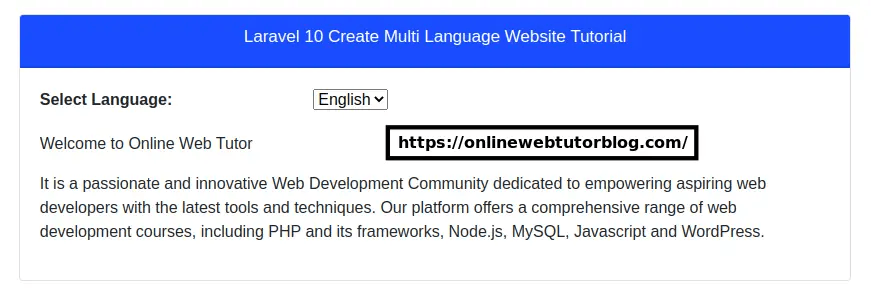
Then this is what it looks like when the Laravel website is translated into Arabic.
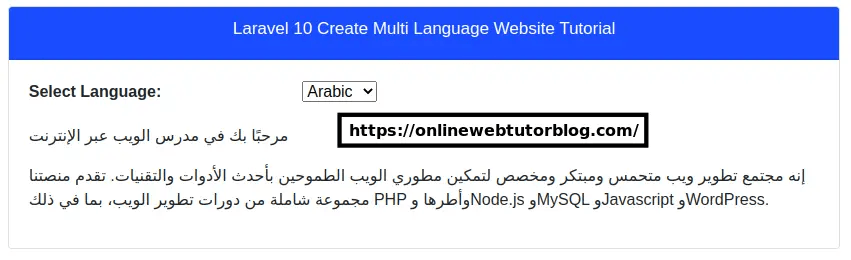
What is the difference between implement Google Cloud AI translation & Google Translate on Laravel?
After understanding each implementation of Google Cloud AI and Google Translate translation in Laravel, we will discuss their differences.
Installation and Configuration Process
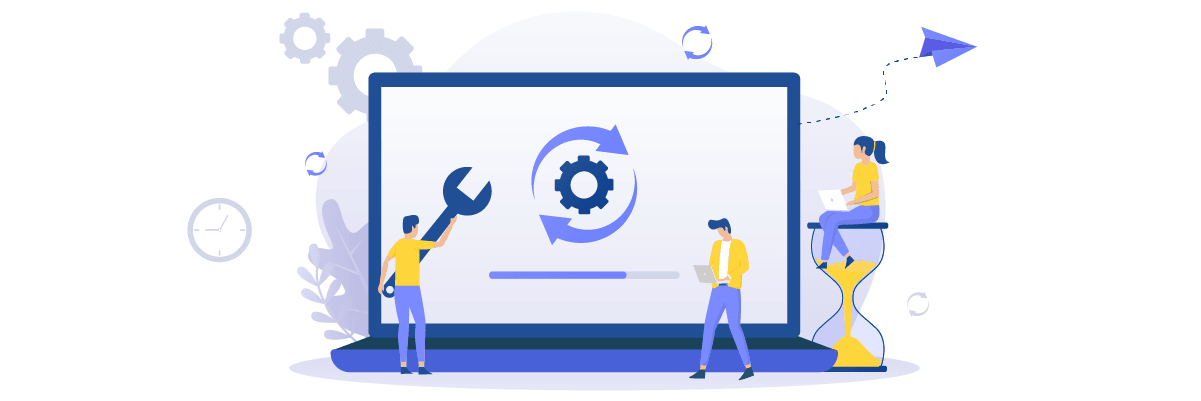
- Google Cloud AI API translation: The installation and configuration process is more complex because you must create a project on Google Cloud Platform (GCP), enable the Cloud Translation API, create a service account, generate API keys, and configure the API client in your Laravel application. This involves several steps and requires a valid GCP account.
- Google Translate: Installation and configuration are much simpler. You only need to install a third-party package or library via Composer, such as stichoza/google-translate-php. After that, you can configure the Google Translate API key in the Laravel configuration file.
Translation Accuracy
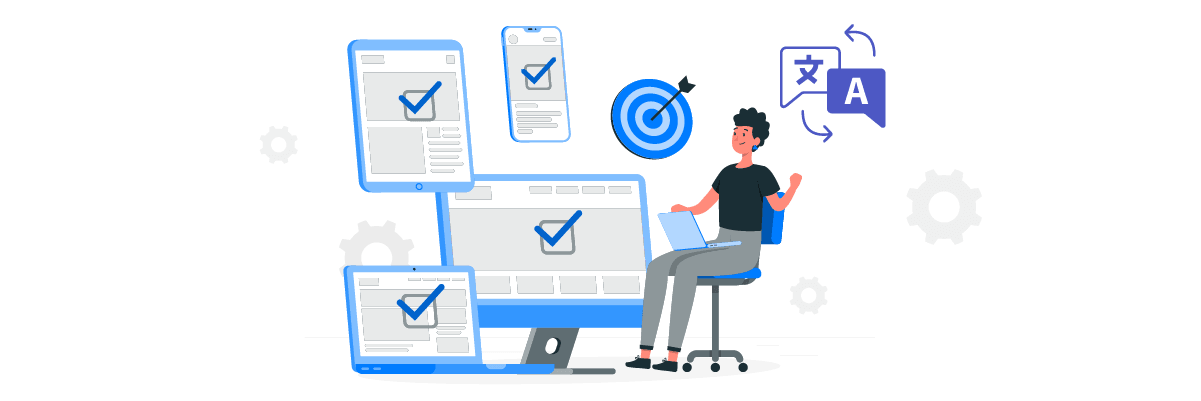
- Google Cloud AI API Translation: Thanks to its deep learning capabilities, this service can better capture complex language contexts and nuances. This allows for more accurate translation of idiomatic expressions, figurative phrases, and wordplays that are difficult to translate literally.
- Google Translate: often struggles to capture finer language context and nuances, making the results sound stiff or less natural. Due to the statistical approach, translating idiomatic expressions or figurative phrases is often less accurate.
Response Speed
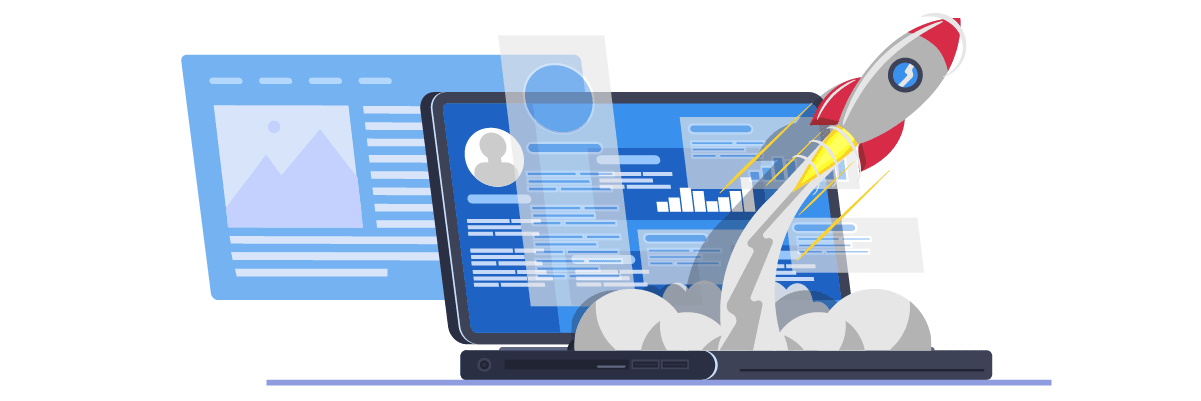
- Google Cloud AI API translation: Generally faster in responding to translation requests as it uses optimized neural machine translation models hosted on Google’s robust cloud infrastructure. This provides quicker response times, especially for longer texts or large volumes of translations.
- Google Translate: It can be slightly slower in responding to translation requests, particularly for longer documents or texts. This is because It uses a more traditional mix of statistical and neural translation approaches, which may not be as efficient as the neural models used by Google Cloud AI API translation.
Customization and Control Capabilities
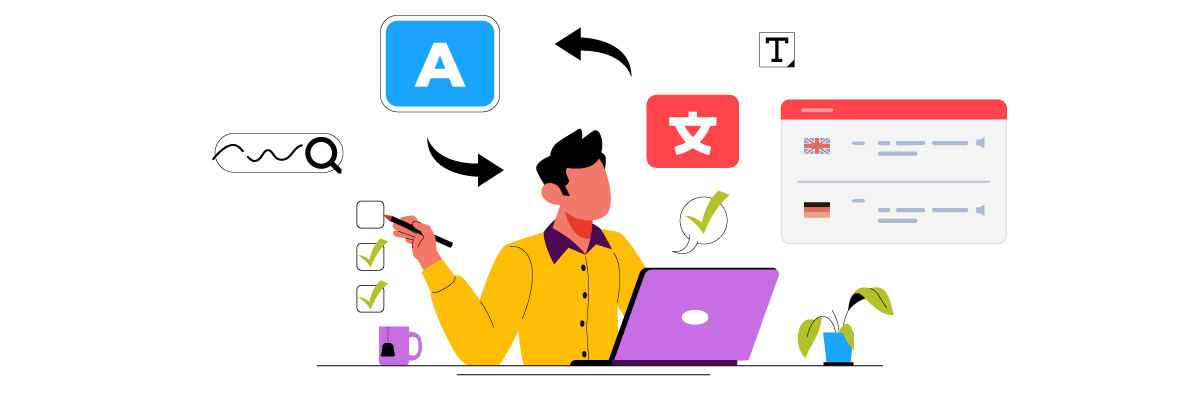
- Google Cloud AI API Translation: Offers more options to customize and control the translation process, such as setting translation preferences, uploading custom glossaries, and accessing the live editor translation interface to edit translation results manually. This allows users to improve translation accuracy according to their needs.
- Google Translate: Has more limited customization and control options. Users must rely on automatic translation results without much ability to edit or directly customize the outcomes.
Advanced Features
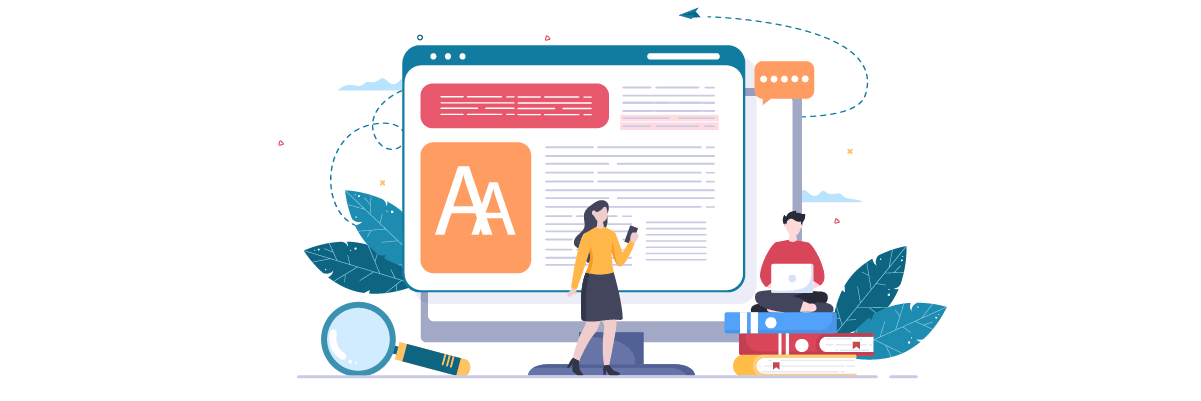
- Google Cloud AI API translation: The Google Cloud AI translation technology has several advanced features such as Glossary, Model selection, and AutoML models. Additionally, in the context of cloud AI translation with Linguise, it also has advanced features like translation exclusions for specific words such as technical terms, brand names, etc., based on lines, pages, or URLs.
- Google Translate: Google Translate installed in Laravel cannot translate audio documents or others. It can only translate text on the website into another language without the ability to edit the translation results.
Factors should you consider when choosing a translation service for your Laravel website
Here are some considerations in choosing the best translation service for your Laravel website.
- High Translation Accuracy – One of the most important factors is the accuracy of the translation produced. If your website requires highly accurate translations, especially for complex content and nuanced language, then Google Cloud AI API Translation might be a better choice than Google Translate.
- Ease of Integration and Configuration — Consider installing and configuring the translation service with your Laravel application. Google Translate is generally easier to integrate because it is available as a PHP package or library. Meanwhile, Google Cloud AI API Translation requires more complex configuration on the Google Cloud Platform.
- Features and Customization Options – If you need additional features such as a glossary or specialized terminology translation, editor translation results, or other customization options, Google Cloud AI API Translation offers more choices than Google Translate.
- Speed and Response Time – For applications with large translation volumes or needing fast response, Google Cloud AI API Translation generally responds to translation requests faster than Google Translate.
- Cost and Budget – Google Translate is available for free for non-commercial use, while Google Cloud AI API Translation uses a paid billing model based on usage. Consider your budget and estimated translation volume to choose the most cost-effective service.
- Support and Documentation – Also, consider the technical support and documentation available for each service. Google Cloud AI API Translation may have more comprehensive documentation and support from Google.
Linguise, the best cloud AI translation service for Laravel website
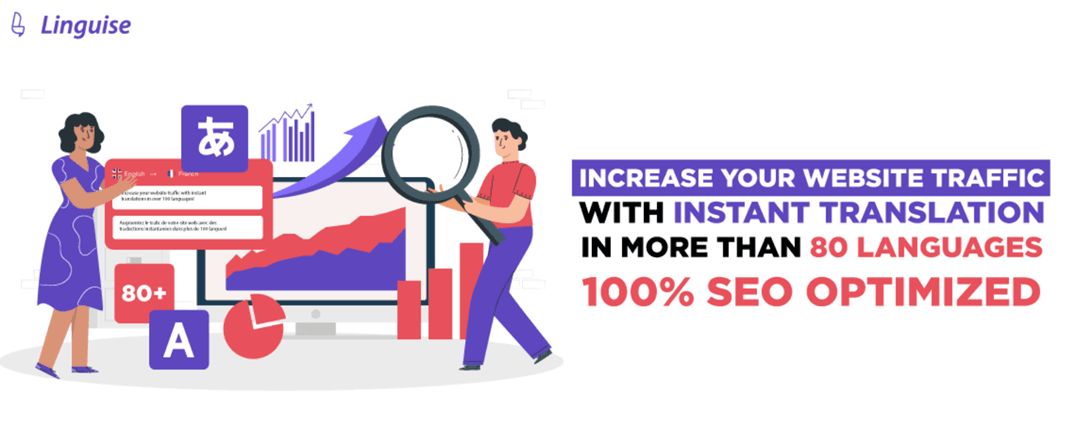
Up to this point, you already know the considerations for choosing a translation service for Laravel; almost all of the factors mentioned above are present in the Linguise translation service.
Linguise is a website translation service using cloud AI translation technology to produce content translations. This service can be the best solution for translating a Laravel-based website. Why is that? Because Linguise addresses the considerations mentioned above.
- Linguise has perfect translation quality. Although this accuracy varies depending on the language pair, Linguise boasts an accuracy rate of nearly 97%, similar to human translation.
- Linguise is also integrated with more than 40 CMS and web builders, as well as integrated with PHP-based websites like Laravel.
- Although it translates automatically, Linguise has a live editor that can be used to edit translation results. This way, the translation can be adjusted to business preferences.
- The loading time for multilingual pages is only 5% longer than that of the original language pages.
- Our service is available in three packages ranging from $15 to $45 per month. Although it is paid, we also offer a free trial period of 30 days with a limit of 600,000 words that can be translated.
- Finally, Linguise supports all forms of customer assistance, including guide articles, documentation, product news, YouTube video tutorials, and 24/7 support chat.
From the points above, Linguise can be a cloud AI translation technology solution for Laravel.
Conclusion
In this article, we have explored the main differences between implementing Google Cloud AI API Translation and Google Translate for Laravel web applications.
Google Cloud AI API Translation excels in accuracy and the need for high-accuracy translations. However, this service requires a more complex configuration and is more expensive than Google Translate. On the other hand, Google Translate offers ease of integration but has limitations in accuracy and customization options.
Linguise emerges as an alternative cloud AI-based translation service compatible with Laravel websites. With accuracy levels nearly matching human translations, translation editor options, fast loading times, and comprehensive customer support, Linguise becomes an excellent translation solution for your Laravel website.
If you want to try Linguise, you can sign up for a free account and enjoy a 30-day trial period with a limit of 600,000 translated words. Visit linguise.com for more information!